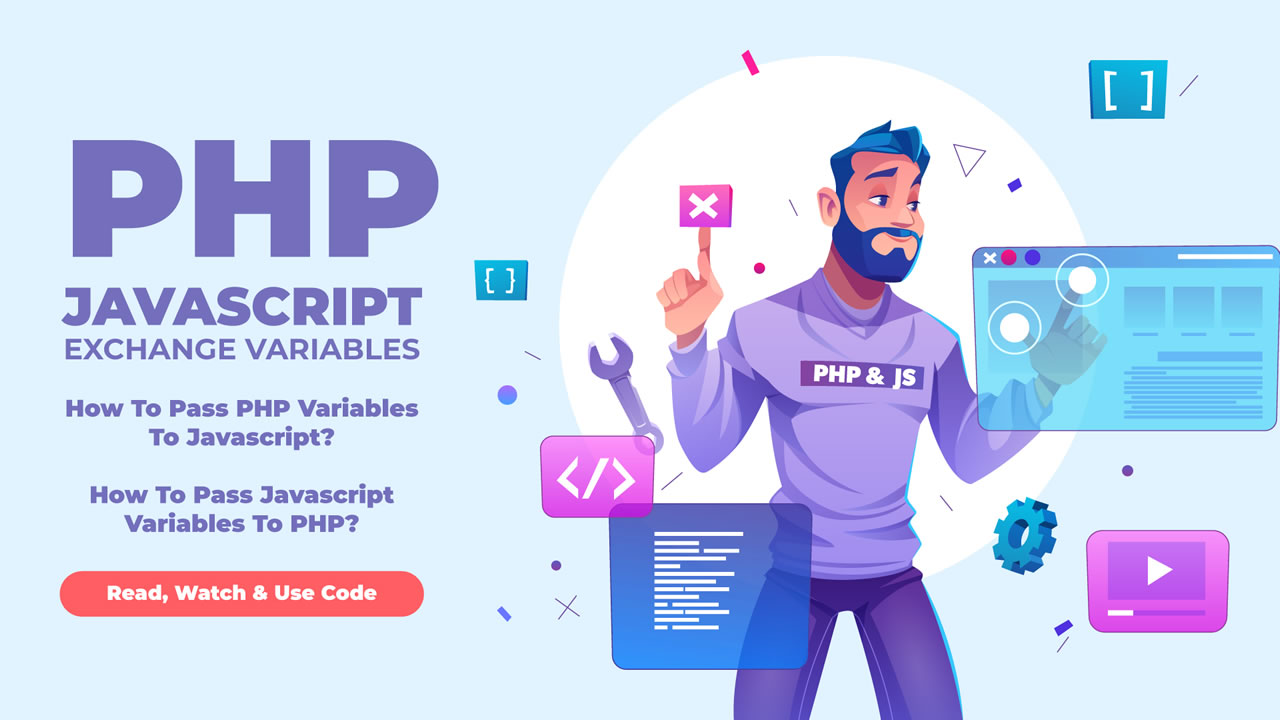
In this tutorial, we will learn how to pass variables from and to PHP and JavaScript. using 6 methods. 2 for passing JavaScript to PHP and 4 methods for passing PHP to JavaScript.
First: How To Pass JavaScript Variables To PHP?
Method 1: Pass the JavaScript variable by Echoing the Script Tag In the PHP File.
In Another JavaScript File:
<script>
var javascript_variable = "success";
</script>
In PHP File:
<?php
$javascript_to_php_variable = echo "<script>document.writeln(javascript_variable);</script>" ;
echo $javascript_to_php_variable;
?>
Method 2: Passing JavaScript variables Using Cookies.
I will use the js-cookie library.
You can see this library documentation on the js-cookie library GitHub: at this link: https://github.com/js-cookie/js-cookie
First, you need to import the CDN link before your JavaScript code., and I want to mention that this library is not a jQuery plugin but it is recommended to put it after the jQuery script tag and before your custom code script tag.
<script src="https://cdnjs.cloudflare.com/ajax/libs/js-cookie/3.0.1/js.cookie.min.js"></script>
In Another JavaScript File: (I will use jQuery)
<script>
$(document).ready(function() {
Cookies.set('cookie_name', 'cookie_value', { expires: 365 });
Cookies.get('cookie_name'); // => 'value'
Cookies.remove('cookie_name');
});
</script>
of course, you would not remove the cookie but I wrote all cookie manipulation statements using the js-cookie library. for your reference.
In PHP File:
<?php
$javascript_to_php_variable = $_COOKIE[$cookie_name];
echo javascript_to_php_variable;
?>
Second: How To Pass PHP Variables To JavaScript?
Method 1: Pass a set of PHP variables using PHP json_encode() function.
In PHP File:
<?php
$php_array = array("key1" => "value1", "key2" => "value2");
<script>
var javascript_object_from_php = <?php echo json_encode($php_array ) ?>;
</script>
?>
In Another JavaScript File: (I will use jQuery), I will set the content of the body to this string:
<script>
$(document).ready(function(){
$("body").text("key1 value:" + javascript_object_from_php['key1'] + ", key2 value: " + javascript_object_from_php['key2']);
});
</script>
Method 2: Pass PHP variable using data attribute.
In PHP File:
<div class="php-variable-container d-none" data-php_variable="<?php echo $php_variable; ?>"></div>
You can add to the div tag the display property and set it to none in the CSS of the class to hide the div tag, I used the bootstrap class d-none. because I use bootstrap in all of my projects.
In Another JavaScript File: (I will use jQuery)
<script>
$(document).ready(function() {
var php_variable_container = $('.php-variable-container');
var php_variable_passed_to_js= php_variable_container.data('php_variable');
});
</script>
Method 3: Pass PHP variable Using Cookies.
In PHP File:
<?php
$cookie_name = "John Doe";
$_COOKIE[$cookie_name];
?>
Import The JS-Cookie Library:
<script src="https://cdnjs.cloudflare.com/ajax/libs/js-cookie/3.0.1/js.cookie.min.js"></script>
In Another JavaScript File: (I will use jQuery), of course, you would not set the cookie before you take its value using the get function of the JS-Cookie library, but I wrote all cookie manipulation statements using this Library. for your reference.
<script>
$(document).ready(function() {
Cookies.set('cookie_name', 'cookie_value', { expires: 365 });
Cookies.get('cookie_name'); // => 'value'
Cookies.remove('cookie_name');
});
</script>
Method 4: Passing HTML Form values on a JSON Using (JSON.stringify() And serializeArray()) jQuery Functions
In PHP File:
<form onsubmit='return onSubmit(this)'>
<input name='user' placeholder='user'><br>
<input name='password' type='password' placeholder='password'><br>
<button type='submit'>Try</button>
</form>
In Another JavaScript File: (I will use jQuery)
You can read the output of serializing array function, using nested jQuery (each) loop function.
<script>
$(document).ready(function() {
function onSubmit( form ){
var data = JSON.stringify($(form).serializeArray());
console.log( data );//[{"name":"user","value":"emad"},{"name":"password","value":"123456"}]
$(data).each(function (index, value) {
$.each(value, function (variable_name, variable_value) {
console.log(variable_name + " : " + variable_value);
});
});
return false; //don't submit
}
});
</script>
That’s it, we learned all the major methods of exchanging variables between the back-end language PHP and the front-end language JavaScript.
Feel Free to bookmark my website to keep it as a reference for your work in the future.
posted by Emad Zedan on 17 Mar 2022 in Web Design, WordPress
Every weekend I used to pay a visit this website, for the reason that I want enjoyment, for the reason that this this web page contains truly nice funny stuff too.
Saved as a favorite, I really like your website!
I’ve learn several good stuff here. Certainly value bookmarking for revisiting. I surprise how much attempt you place to create the sort of magnificent informative website.
Fɑbᥙlous, what a website it is! This blog ρresents һelpful facts to us, keep it up.
This piece of writing will assist the internet users for setting up new webpage or even a weblog from start to end.
Hi there to all, it’s actually a fastidious for me to go to see this web page, it consists of precious Information.
Right here is thee right blog for anybody who would like to understand this topic. You realize a whole lot its almost tough to argue with you (not that I personally would want to… haha). You certainly put a new spin on a subject that’s been written about for ages. Great stuff, just excellent!
Bookmarked!!, I love your web site!