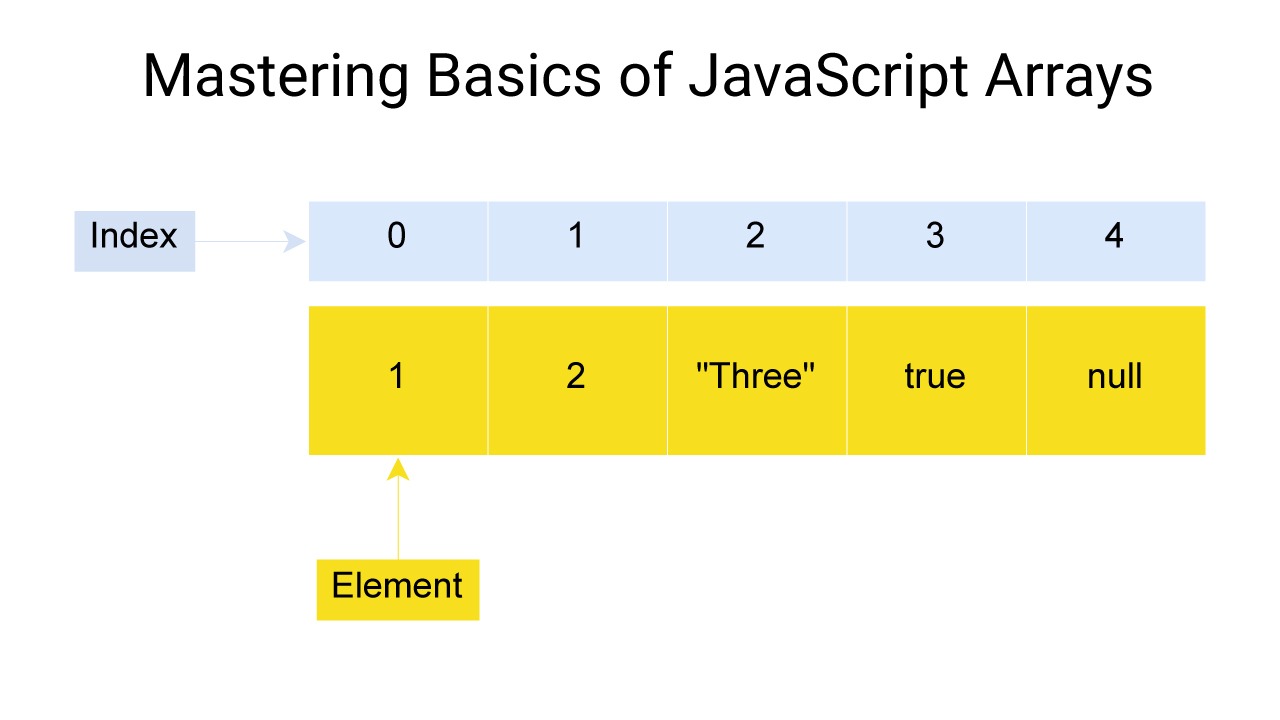
JavaScript arrays are fundamental building blocks for storing and manipulating ordered collections of elements. They offer a powerful way to group various data types, from simple numbers and strings to complex objects and even other arrays. This guide delves into the world of JavaScript arrays, exploring their creation, manipulation, and essential functions.
Creating JavaScript Arrays
There are two primary ways to create JavaScript arrays:
Array Literal: The most common approach is using square brackets [] and listing elements separated by commas.
const fruits = ["apple", "banana", "orange"];
const numbers = [1, 2, 3, 4, 5];
Array Constructor: The Array() constructor can also be used, but it’s generally less preferred for simple arrays.
const vegetables = new Array("potato", "carrot", "broccoli");
Essential JavaScript Array Functions
JavaScript provides a rich set of functions to interact with arrays effectively. Let’s explore some of the most commonly used ones:
Accessing Elements: Use the index within square brackets [] to access individual elements. Arrays are zero-indexed, meaning the first element has an index of 0.
const colors = ["red", "green", "blue"];
console.log(colors[1]); // Outputs: "green"
.length Property: The length property returns the number of elements in the array.
const animals = ["cat", "dog", "bird"];
console.log(animals.length); // Outputs: 3
.push(): The .push() method adds one or more elements to the end of the array and returns the new length.
const groceries = ["milk", "bread"];
groceries.push("eggs");
console.log(groceries); // Outputs: ["milk", "bread", "eggs"]
.pop(): The .pop() method removes and returns the last element from the array.
const planets = ["Earth", "Mars", "Jupiter"];
planets.pop();
console.log(planets); // Outputs: ["Earth", "Mars"]
.shift(): The .shift() method removes and returns the first element from the array.
const countries = ["France", "Germany", "Italy"];
countries.shift();
console.log(countries); // Outputs: ["Germany", "Italy"]
.unshift(): The .unshift() method adds one or more elements to the beginning of the array and returns the new length.
const languages = ["JavaScript", "Python"];
languages.unshift("Java");
console.log(languages); // Outputs: ["Java", "JavaScript", "Python"]
.indexOf(): The .indexOf() method searches the array for a specific element and returns its index. If the element is not found, it returns -1.
const numbers = [10, 20, 30, 40];
console.log(numbers.indexOf(30)); // Outputs: 2
.slice(): The .slice() method extracts a section of the array and returns a new array. It takes two arguments: the starting index (inclusive) and the ending index (exclusive).
const alphabet = ["a", "b", "c", "d", "e"];
const slicedAlphabet = alphabet.slice(1, 4);
console.log(slicedAlphabet); // Outputs: ["b", "c", "d"]
.concat(): The .concat() method merges two or more arrays and returns a new array.
const fruits = ["apple", "banana"];
const vegetables = ["potato", "carrot"];
const allProduce = fruits.concat(vegetables);
console.log(allProduce); // Outputs: ["apple", "banana", "potato", "carrot"]
.join(): The .join() method joins all elements of an array into a string, separated by a specified separator (comma by default).
const colors = ["red", "green", "blue"];
console.log(colors.join()); // Outputs: "red,green,blue"
console.log(colors.join(" - ")); // Outputs: "red - green - blue"
These are just a few of the many powerful JavaScript array functions available. By understanding and utilizing these functions, you can effectively manage and manipulate data within your JavaScript applications.
Conclusion
JavaScript arrays offer a versatile and efficient way to store and manage collections of elements. Their flexibility allows you to hold various data types and manipulate them using a rich set of built-in functions. This guide provided a foundation for understanding JavaScript arrays. As you delve deeper into JavaScript programming, explore additional functionalities like:
- .forEach(): Executes a provided function once for each array element.
- .map(): Creates a new array with the results of calling a function on every element in the array.
- .filter(): Creates a new array with elements that pass a test implemented by the provided function.
- .reduce(): Applies a function against an accumulator and each element in the array to reduce it to a single value.
By mastering these core concepts and functions, you’ll unlock the full potential of JavaScript arrays, enabling you to build dynamic and interactive web applications.
posted by Emad Zedan on 07 Jun 2024 in Coding, Development, Web Design, WordPress
Leave a Reply